方法
這裡列出了進階 API 的方法,包括初始化、掛載、更新外觀、更新語言和結帳等。
createPayment
用法
ts
PayNow.createPayment({
publicKey: 'pk_xxxxxxxxxxxxx',
secret: 'sk_xxxxxxxxxxxxx',
env: 'sandbox'
})
型別定義
ts
interface CreatePaymentOptions {
/**
* The public key of your account in PayNow
*/
publicKey: string,
/**
* The secret of every payment request that you create by using the secret key to sign the request
*/
secret: string,
/**
* environment definition
*
* @default 'production'
*/
env: EnvironmentEnum
}
mount
用法
ts
PayNow.mount('#paynow-container', {
locale: 'zh_tw',
appearance: {
variables: {
colorPrimary: '#ff7800',
},
styles: {
'.PN_FormInput': {
padding: '1rem',
borderRadius: '0.5rem',
}
}
},
paymentMethods: ['CreditCard', 'CreditCardInstallment', 'ATM', 'ApplePay', 'ConvenienceStore']
})
型別定義
ts
interface MountOptions {
/**
* HTML element or selector, e.g. '#paynow-container'
*/
el: HTMLElement | string,
options?: {
/**
* interface language
*
* @default 'en'
*/
locale: LocaleEnum,
/**
* override styles
*/
appearance?: AppearanceOptions,
/**
* define the methods you want to provide to your users (including the sequence) if its available
*/
paymentMethods?: PaymentMethodsEnum[]
}
}
回呼函式
ts
PayNow.on('mounted', () => {
// handle callback
})
updateLocale
用法
ts
PayNow.updateLocale('zh_tw')
型別定義
ts
type LocaleEnum = 'zh_tw' | 'en' | 'ja' | 'zh_cn'
回呼函式
ts
PayNow.on('localeUpdated', (locale) => {
// handle callback
})
updateAppearance
用法
ts
PayNow.updateAppearance({
theme: 'light',
variables: {
colorPrimary: '#078abc',
},
styles: {
'.PN_FormInput': {
padding: '0.5rem 1rem',
borderRadius: '1rem',
}
}
})
樣式選擇器
Class 名稱 | 說明 |
---|---|
.PN_Container | 付款表單的容器 |
.PN_FormMethodOption | 付款方式選項 |
.PN_FormMethodOption--selected | 已選擇的付款方式選項 |
.PN_FormMethodMore | 更多選項按鈕 |
.PN_FormCodeType | 便利商店的代碼類型選項 |
.PN_FormCodeType--selected | 已選擇的便利商店代碼類型選項 |
.PN_FormInstallment | 分期付款選項 |
.PN_FormInstallment--selected | 已選擇的分期付款選項 |
.PN_FormLabel | 輸入欄位的標籤 |
.PN_FormInput | 輸入欄位 |
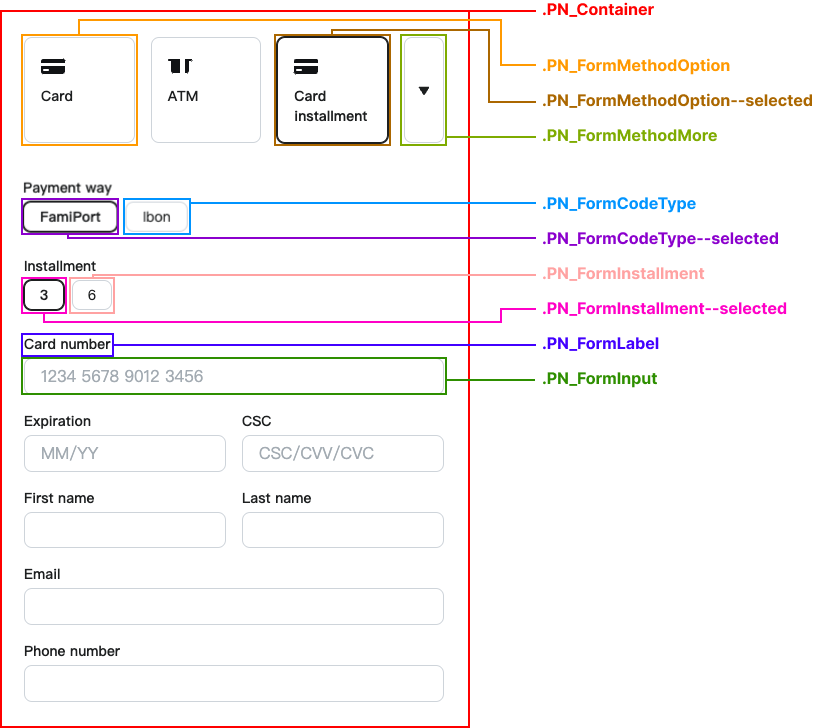
型別定義
ts
type AppearanceOptions = {
/**
* @default 'light'
*/
theme?: 'light' | 'dark' | 'limegreen' | 'desert',
variables?: {
colorPrimary: string,
colorBorder: string,
colorPlaceholder: string,
borderRadius: string,
colorDefault: string,
colorDanger: string,
},
styles?: {
[selector: string]: {
[property: string]: string;
}
}
}
checkout
用法
ts
PayNow.checkout().then((response: CheckoutResponse) => {
if ('error' in response) {
// handle error
}
// handle success
})
型別定義
ts
type FieldErrorType = {
error: {
message: string, // 'Please complete the form'
fields: {
/**
* will return card type when field name is 'CARD_NUMBER', e.g., 'visa'
*/
cardType?: string,
/**
* field value is valid
*/
isComplete: boolean,
/**
* error message if status is invalid, e.g., 'Invalid card number'
*/
message: string,
/**
* field name, e.g., 'CARD_NUMBER'
*/
name: string,
status: FieldErrorStatus,
/**
* will return the values of inputs other than card information.
*/
value?: string
}[]
}
}
enum FieldErrorStatus {
/**
* field is valid
*/
VALID = 0,
/**
* field is invalid in some rules
*/
INVALID = 1,
/**
* field is required, but got empty
*/
INCOMPLETE = 2
}
type ResponseErrorType = {
error: {
message: string,
status: string,
type: string,
result: string
}
}
type ATMResponseType = {
bankBranchCode: string,
bankBranchName: string,
bankCode: string,
bankName: string,
expiredAt: string,
virtualAccountNo: string
}
type ConvenienceStoreResponseType = {
codeType: string,
expiredAt: string,
payCodeNo: string,
qrCode: string
}
type DefaultResponse = {
allowPaymentMethodTypes: string[],
amount: number,
createdAt: string,
currency: string,
description: string,
id: string,
payment: {
amount: number,
createdAt: string,
currency: string,
description: string,
meta: {
approvalCode: string,
cardType: string,
installments: number | null,
lastFourDigitsOfCard: string,
respCode: string
},
paidAt: string,
payerEmail: string,
payerName: string,
paymentMethod: string,
paymentNo: string,
paymentToken: string,
products: {
name: string,
description: string,
price: number,
currency: string,
quantity: number
}[],
redirectUrl: string,
source: string,
status: string,
transactionNo: string
},
secret: string,
status: string,
}