Methods
Methods that can be called on the PayNow instance.
createPayment
Usage
ts
PayNow.createPayment({
publicKey: 'pk_xxxxxxxxxxxxx',
secret: 'sk_xxxxxxxxxxxxx',
env: 'sandbox'
})
Type declarations
ts
interface CreatePaymentOptions {
/**
* The public key of your account in PayNow
*/
publicKey: string,
/**
* The secret of every payment request that you create by using the secret key to sign the request
*/
secret: string,
/**
* environment definition
*
* @default 'production'
*/
env: EnvironmentEnum
}
mount
Usage
ts
PayNow.mount('#paynow-container', {
locale: 'zh_tw',
appearance: {
variables: {
colorPrimary: '#ff7800',
},
styles: {
'.PN_FormInput': {
padding: '1rem',
borderRadius: '0.5rem',
}
}
},
paymentMethods: ['CreditCard', 'CreditCardInstallment', 'ATM', 'ApplePay', 'ConvenienceStore']
})
Type declarations
ts
interface MountOptions {
/**
* HTML element or selector, e.g. '#paynow-container'
*/
el: HTMLElement | string,
options?: {
/**
* interface language
*
* @default 'en'
*/
locale: LocaleEnum,
/**
* override styles
*/
appearance?: AppearanceOptions,
/**
* define the methods you want to provide to your users (including the sequence) if its available
*/
paymentMethods?: PaymentMethodsEnum[]
}
}
Callback
ts
PayNow.on('mounted', () => {
// handle callback
})
updateLocale
Usage
ts
PayNow.updateLocale('zh_tw')
Type declarations
ts
type LocaleEnum = 'zh_tw' | 'en' | 'ja' | 'zh_cn'
Callback
ts
PayNow.on('localeUpdated', (locale) => {
// handle callback
})
updateAppearance
Usage
ts
PayNow.updateAppearance({
theme: 'light',
variables: {
colorPrimary: '#078abc',
},
styles: {
'.PN_FormInput': {
padding: '0.5rem 1rem',
borderRadius: '1rem',
}
}
})
Style selectors
Class name | Description |
---|---|
.PN_Container | The container of the payment form |
.PN_FormMethodOption | The payment method option |
.PN_FormMethodOption--selected | The selected payment method option |
.PN_FormMethodMore | The more option button |
.PN_FormCodeType | The code type option of convenience store |
.PN_FormCodeType--selected | The selected code type option of convenience store |
.PN_FormInstallment | The installment option |
.PN_FormInstallment--selected | The selected installment option |
.PN_FormLabel | The label of the input field |
.PN_FormInput | The input field |
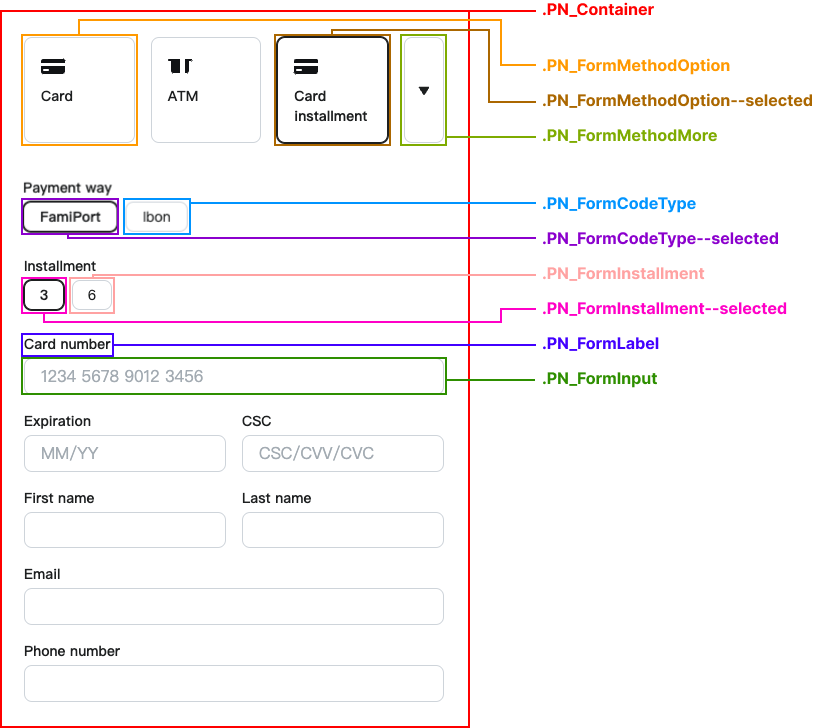
Type declarations
ts
type AppearanceOptions = {
/**
* @default 'light'
*/
theme?: 'light' | 'dark' | 'limegreen' | 'desert',
variables?: {
colorPrimary: string,
colorBorder: string,
colorPlaceholder: string,
borderRadius: string,
colorDefault: string,
colorDanger: string,
},
styles?: {
[selector: string]: {
[property: string]: string;
}
}
}
checkout
Usage
ts
PayNow.checkout().then((response: CheckoutResponse) => {
if ('error' in response) {
// handle error
}
// handle success
})
Type declarations
ts
type FieldErrorType = {
error: {
message: string, // 'Please complete the form'
fields: {
/**
* will return card type when field name is 'CARD_NUMBER', e.g., 'visa'
*/
cardType?: string,
/**
* field value is valid
*/
isComplete: boolean,
/**
* error message if status is invalid, e.g., 'Invalid card number'
*/
message: string,
/**
* field name, e.g., 'CARD_NUMBER'
*/
name: string,
status: FieldErrorStatus,
/**
* will return the values of inputs other than card information.
*/
value?: string
}[]
}
}
enum FieldErrorStatus {
/**
* field is valid
*/
VALID = 0,
/**
* field is invalid in some rules
*/
INVALID = 1,
/**
* field is required, but got empty
*/
INCOMPLETE = 2
}
type ResponseErrorType = {
error: {
message: string,
status: string,
type: string,
result: string
}
}
type ATMResponseType = {
bankBranchCode: string,
bankBranchName: string,
bankCode: string,
bankName: string,
expiredAt: string,
virtualAccountNo: string
}
type ConvenienceStoreResponseType = {
codeType: string,
expiredAt: string,
payCodeNo: string,
qrCode: string
}
type DefaultResponse = {
allowPaymentMethodTypes: string[],
amount: number,
createdAt: string,
currency: string,
description: string,
id: string,
payment: {
amount: number,
createdAt: string,
currency: string,
description: string,
meta: {
approvalCode: string,
cardType: string,
installments: number | null,
lastFourDigitsOfCard: string,
respCode: string
},
paidAt: string,
payerEmail: string,
payerName: string,
paymentMethod: string,
paymentNo: string,
paymentToken: string,
products: {
name: string,
description: string,
price: number,
currency: string,
quantity: number
}[],
redirectUrl: string,
source: string,
status: string,
transactionNo: string
},
secret: string,
status: string,
}